Top tools for API testing in 2020
What is an API
An Application Programming Interface (API) is a layer of communication that facilitates the exchange of data between the various components in a software application. APIs are playing an ever more important role in web development because the different parts of a web application may be built as standalone micro-services that communicate as a whole through such designated interfaces.
The API layer usually describes a set of rules that define the expected behavior when interacted with by a component requesting for data, these rules help in validating the response to ensure that things always work as they should.
What is API testing
APIs are becoming the preferred method of communication between web components and this has the effect of driving the business logic farther into their implementation. This means that APIs need to be tested to catch and re-mediate bugs before they reach production.
API testing is a type of software testing that directly tests the application programming interface to determine if it correctly responds to requests, meets functionality, performance and security standards.
API testing is sometimes called “headless” testing because it usually tests the behavior of a User interface (UI) without actually interacting with or loading the UI, but by sending requests directly to the backend API.
Benefits of API testing
API testing promises several benefits that can impact the efficiency of the test suite positively. A notable benefit of API testing is that it is capable of quickly catching bugs in the test cycle, and this is useful in giving quick feedback to developer teams.
API tests are also a better choice when compared to UI tests as the latter can be slow to implement and can result in flaky results.
Some awesome free tools for API testing
There are several ways to go about API testing, one of which is directly writing code in your preferred language to hit the endpoints and confirm that the response and data are in-line with expectations. As with everything else in software development, there are dedicated tools to automate the process and make things significantly easier and faster.
We are going to look at some awesome tools that simplify the process of API testing and make it easy to reuse the same tests with different API services.
RestAssured
REST Assured is a Java library that provides a domain-specific language (DSL) for writing powerful, maintainable tests for RESTful APIs. It makes testing easy by eradicating the need for boilerplate code while validating complex responses.
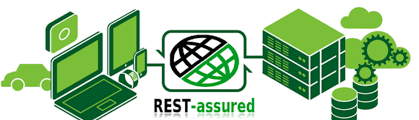
Here are some of its features and benefits:
It supports XML and JSON Request/Responses.
It supports BDD Given/When/Then syntax.
It Integrates seamlessly with Java projects.
If you want to learn more about REST Assured, take a look at the usage guide on GitHub.
Loadmill
Loadmill is a test service that supports API testing by recording real user sessions in your application and converting them to reliable API tests. It saves time and effort because it eliminates the process of scripting your tests manually.
The process of generating these recordings is fast and only takes a few minutes.
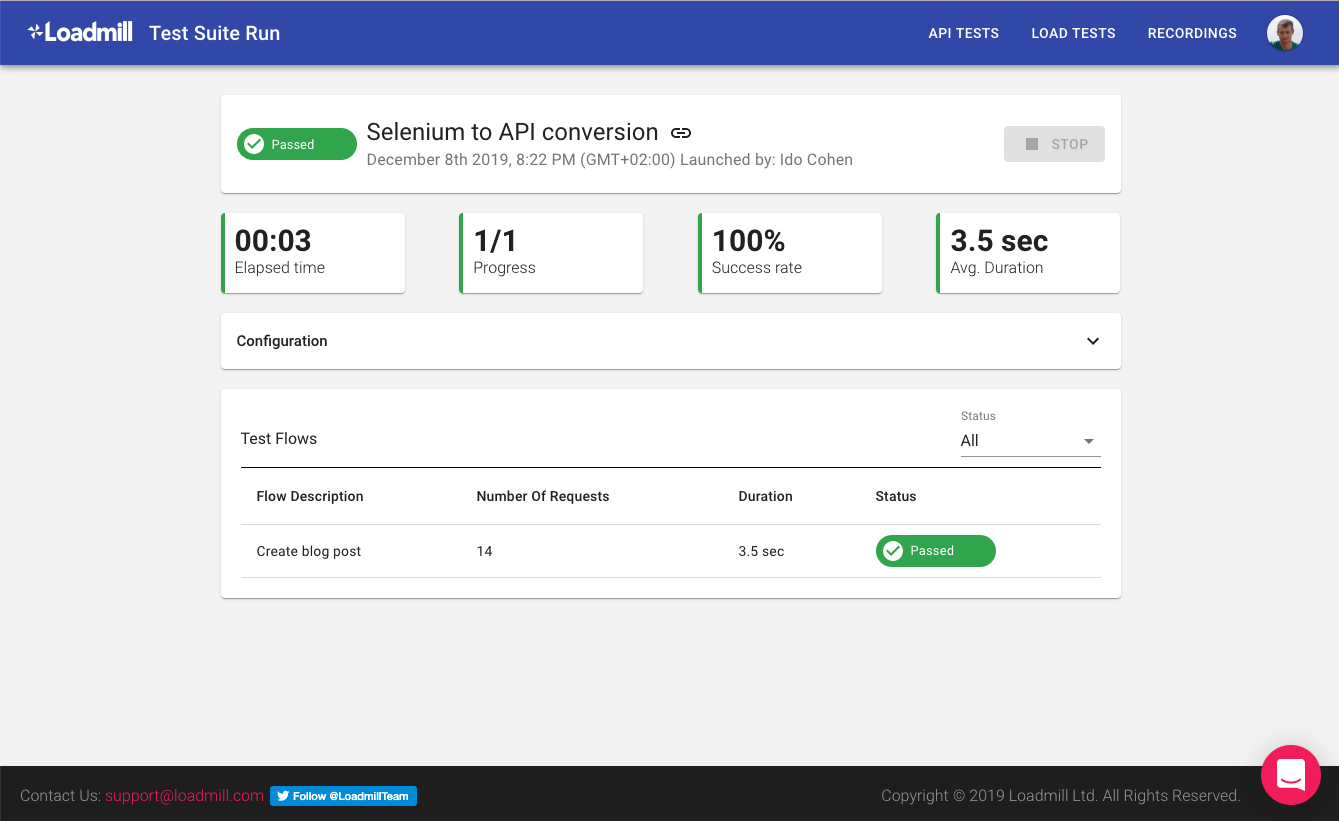
Here are some benefits of Loadmill:
It automatically generates regression tests from real user recordings.
It ensures that you move fast and your focus is on what matters.
It automates the QA process by replaying real user behavior.
It ships with an easy to use and interact with user interface.
If you want to learn more about LoadMill, take a look at their GitHub.
RestSharp
RestSharp is a popular HTTP client library for .NET. featuring automatic serialization and deserialization, request and response type detection, variety of authentications and other useful features. It supports the following features:
Supports GET, POST, PUT, PATCH, HEAD, OPTIONS, DELETE request options.
Easy installation with NuGet for most .NET flavours.
Supports .NET 3.5+, Silverlight 5, Windows Phone 8, Mono, MonoTouch, Mono for Android
To find out more about RestShare, check out the official repository here.
Postman
Postman is one of the more popular tools for testing REST services. It started as a Chrome browser plugin but now has dedicated native versions that developers and testers alike rely on heavily to check API connections and services.
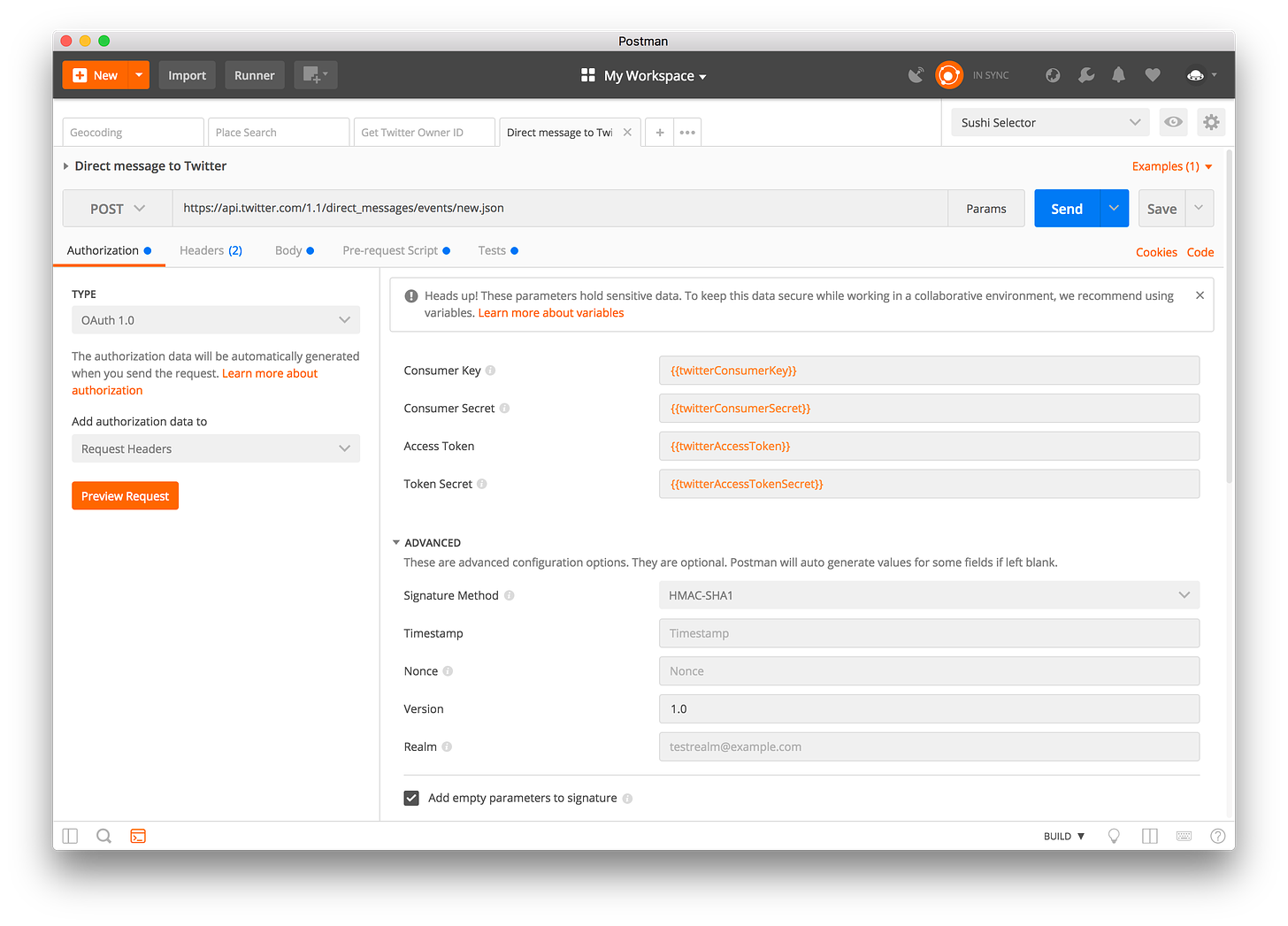
Postman can be used for various levels of API testing, a common way to perform a test is to send a POST request to the API endpoints to be tested and check that the responses are in-line with expectations. It also allows you to set headers and cookies for authentication and data parsing.
It has three plans:
There is a free plan for individuals and small teams.
There is a Pro plan that supports a team of up to 50 people.
The enterprise plan supports a team of any size and the cost is $18 per user per month.
Here are some benefits associated with using Postman:
Can produce the code representation of an executed test in several languages.
Can run as a browser plugin extension.
Can run as a native application
Doesn't require the knowledge of a new programming language.
Easy to understand UI.
Supports a bunch of integrations like Swagger & RAML formats.
You can find out more about Postman and its use cases by visiting the official website.
Pyresttest
Pyresttest is a Python-based REST testing and API micro-benchmarking tool that supports the following features:
Tests are defined in basic YAML or JSON config files, no code needed.
Minimal dependencies, making it easy to deploy on-server for smoke tests/health checks.
Supports generate/extract/validate mechanisms to create full test scenarios.
Returns exit codes on failure, to slot into automated configuration management/orchestration tools (also supplies parseable logs).
Logic is written and extensible in Python.
It is easy to install and the YAML syntax makes it easy to write. Here is an example test that checks that APIs accept operations, and will smoke-test an application:
---
- config:
- testset: "Basic tests"
- timeout: 100 # Increase timeout from the default 10 seconds
- test:
- name: "Basic get"
- url: "/api/person/"
- test:
- name: "Get single person"
- url: "/api/person/1/"
- test:
- name: "Delete a single person, verify that works"
- url: "/api/person/1/"
- method: 'DELETE'
- test: # create entity by PUT
- name: "Create/update person"
- url: "/api/person/1/"
- method: "PUT"
- body: '{"first_name": "Gaius","id": 1,"last_name": "Baltar","login": "gbaltar"}'
- headers: {'Content-Type': 'application/json'}
- validators: # This is how we do more complex testing!
- compare: {header: content-type, comparator: contains, expected:'json'}
- compare: {jsonpath_mini: 'login', expected: 'gbaltar'} # JSON extraction
- compare: {raw_body:"", comparator:contains, expected: 'Baltar' } # Tests on raw response
- test: # create entity by POST
- name: "Create person"
- url: "/api/person/"
- method: "POST"
- body: '{"first_name": "William","last_name": "Adama","login": "theadmiral"}'
- headers: {Content-Type: application/json}
You can find more details about Pyresttest here.
SoapUI
SoapUI is a functional testing tool for SOAP and rest testing. It ships with an easy-to-use graphical interface and allows you to easily create and execute automated functional, regression and load tests in a single test environment.’
It comes in two versions: Free open source version and the Pro version. Since the free version is open-source, you can tweak the source code to work in a custom way and satisfy your testing needs. Here are some features of the free version:
Supports drag and drop test creating.
Is capable of creating complex test scenarios.
Allows for asynchronous testing.
It has a mock service that lets you mimic web services before the final implementation.
If you find it interesting so far, you can learn more about SoapUI here.
Conclusion
Modern web applications are built as standalone components that communicate through a dedicated Application Programming Interface. This has the effect of shifting the business logic from the UI to the API layer. API testing refers to the implementation and running of tests that assert that an API works as is expected, and sends the correct response codes and data when a valid request is encountered.
In this article, we explored some tools that simplify the process of API testing by offering sleek reusable services that can effectively communicate with REST endpoints, compute intelligible reports when errors are found and lots more.